Exploring the with statement in Python

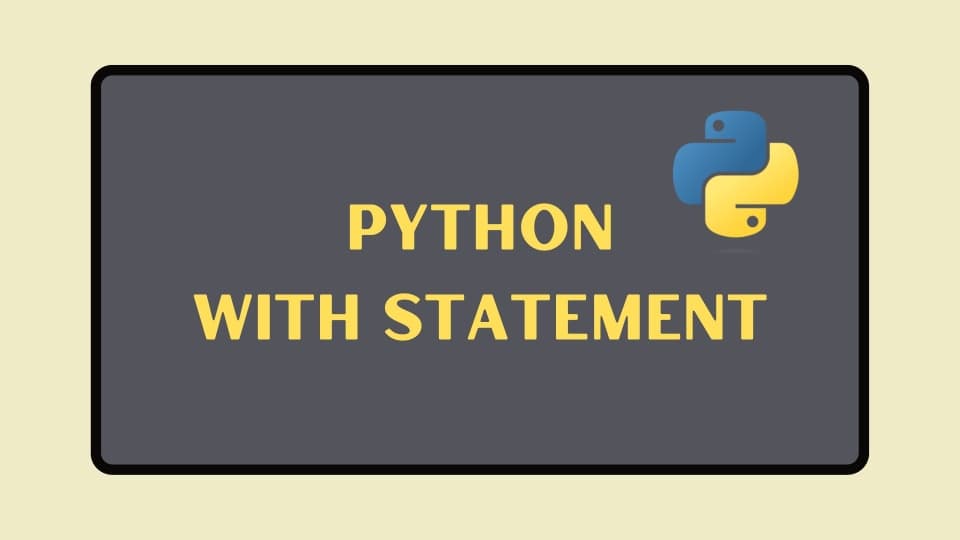
Introduction
In Python, resources such as files, database connections, and network sockets need to be properly managed to avoid leaks and other issues. Managing these resources can be tedious and error-prone, but the with
statement provides an elegant solution to this problem.
The with
statement in Python is a way to wrap a block of code with methods defined by a context manager. A context manager is an object that defines the methods enter() and exit() to perform operations when entering and exiting the context.
with open("myfile.txt", "r") as f
data = f.read()
# do something
ทำความรู้จักกับ with statement
with statement จะมาช่วยให้ code เราอ่านง่ายและ clean มากยิ่งขึ้น ...ก่อนอื่นผมอยากให้ทุกคนดู code ตัวอย่างการอ่านไฟล์ด้านล่างนี้กันก่อนนะครับ
ตัวอย่างการอ่านไฟล์แบบปกติ
f = open("hello.txt", "r")
data = f.read()
# do something
f.close()
code ด้านบนจะมีการเปิดไฟล์ อ่านข้อมูล แล้วก็ do something สุดท้ายด้วยการมาปิดไฟล์ ปัญหาคือถ้าในขั้นตอน do something เกิด Error ไฟล์นี้ก็ยังไม่ถูกปิด ...และถ้าเรารันมันเรื่อยๆ มันก็จะเปิดไม่ปิด เปิดไม่ปิด วนไป
ปัญหานี้ก็แก้ได้ไม่ยาก สร้างเงื่อนไขถ้ามัน Error หรือทำงานเสร็จก็ให้ปิดไฟล์นี้ โดยใช้ try except finally
มาช่วย จะได้ code แบบด้านล่าง
ตัวอย่างการอ่านไฟล์แบบ handle error
f = open("myfile.txt", "r")
try:
data = f.read()
# do something
finally:
f.close()
แต่เพื่อให้อ่านง่ายขึ้นและ code เป็นระเบียบขึ้น เราสามารถใช้ with statement ในการจัดการเหตุการณ์ดังกล่าวแทนได้ จะได้หน้าตาสวยๆดัง code ด้านล่าง
ตัวอย่างการอ่านไฟล์ด้วย with statement
with open("myfile.txt", "r") as f
data = f.read()
# do something
เรามักจะใช้ with เมื่อไร
หลายๆคนที่เคยใช้ python กันมาน่าจะเคยเจอในหลายๆกรณี ผมขอยกตัวอย่างที่เจอได้บ่อยดังนี้
- การอ่าน/เขียนไฟล์
- เชื่อมต่อ database
- การเปิดเว็บของ selenium
เขียน With Statement ใช้เองทำอย่างไร
วิธีที่ 1
ใช้ __enter__
สั่งการทำงานตอนเรียกใช้ with และ __exit__
ทำงานในตอนที่ใช้งานเสร็จหรือเกิด Error
ตัวอย่างการสร้าง with statement ด้วย enter และ exit
class mylib:
def __init__(self, path):
self.path = path
def __enter__(self):
self.file = open(self.path, 'r')
return self.file
def __exit__(self, *args):
self.file.close()
with mylib('myfile.txt') as f:
data = f.read()
วิธีที่ 2
เราสามารถใช้ contextlib
โดยใช้ @contextmanager
แปะไว้บน function ที่เราต้องการใช้งาน เพื่อเปลี่ยนให้มันเป็น with statement ได้แบบนี้เลย
ตัวอย่างการสร้าง with statement ด้วย contextlib
from contextlib import contextmanager
@contextmanager
def mylib(path):
fx = open(path, 'r')
try:
yield fx
finally:
fx.close()
with mylib('myfile.txt') as f:
data = f.read()
Conclusion
The with
statement in Python is a powerful feature that simplifies resource management by automatically handling the opening and closing of files, database connections, network sockets, and other objects. It provides an elegant and concise way to write code that is less prone to errors and avoids resource leaks. If you're not already using the with
statement in your Python code, it's definitely worth exploring.
ติดตามความรู้ Python ได้ที่ DataHungry
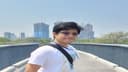
Wuttichai Kaewlomsap
Sr. Data Engineer